struct
Tablo::Border
- Tablo::Border
- Struct
- Value
- Object
Overview
The Border
class enhances the layout of a data table by separating rows
and columns with interconnected horizontal and vertical lines.
Various predefined line types are available, but you are free to create your own.
A border can be styled by a user defined proc, of type Styler
allowing
for colorized output, either by using ANSI sequences or the colorize module
from the stdlib (default: no style).
A border is defined by a string of exactly 16 characters, which is then converted into 16 strings of up to 1 character each. The definition string can contain any character, but two of them have a special meaning: during conversion, the uppercase E is replaced by an empty string, and the uppercase S is replaced by a space (a simple space may also be used, of course).
Please note that using the capital E may cause alignment difficulties.
Examples of text or graphic connectors:
| Name | 16 chars string |
| ------------------------------ | --------------- |
| CONNECTORS_SINGLE_ROUNDED | ╭┬╮├┼┤╰┴╯│││──── |
| CONNECTORS_SINGLE | ┌┬┐├┼┤└┴┘│││──── |
| CONNECTORS_DOUBLE | ╔╦╗╠╬╣╚╩╝║║║════ |
| CONNECTORS_SINGLE_DOUBLE | ╒╤╕╞╪╡╘╧╛│││════ |
| CONNECTORS_DOUBLE_SINGLE | ╓╥╖╟╫╢╙╨╜║║║──── |
| CONNECTORS_HEAVY | ┏┳┓┣╋┫┗┻┛┃┃┃━━━━ |
| CONNECTORS_LIGHT_HEAVY | ┍┯┑┝┿┥┕┷┙│││━━━━ |
| CONNECTORS_HEAVY_LIGHT | ┎┰┒┠╂┨┖┸┚┃┃┃──── |
| CONNECTORS_TEXT_CLASSIC | +++++++++|||---- |
Mixed graphic character sets, such as:
| Name | 16 chars string |
| ------------------------------ | --------------- |
| CONNECTORS_SINGLE_DOUBLE_MIXED | ╔╤╗╟┼╢╚╧╝║│║═─═- |
may not be correctly rendered.
Below is a detailed representation of each ruletype and meaning:
The first 9 characters define the junction or intersection of horizontal and vertical border lines.
Pos Connector name Example (using Fancy border preset)
--- -------------- -----------------------------------
0 top_left "┌"
1 top_mid "┬"
2 top_right "┐"
3 mid_left "├"
4 mid_mid "┼"
5 mid_right "┤"
6 bottom_left "└"
7 bottom_mid "┴"
8 bottom_right "┘"
The next three characters define vertical separators in data rows.
9 vdiv_left "│"
10 vdiv_mid ":"
11 vdiv_right "│"
And finally, the last four characters define the different types of horizontal border, depending on the type of data row or types of adjacent data rows.
12 hdiv_tbs "─" (title or top or bottom or summary)
13 hdiv_grp "−" (group)
14 hdiv_hdr "-" (header)
15 hdiv_bdy "⋅" (body)
Eight predefined borders, of type PreSet
, can also be used instead of
a definition string.
| name | 16 chars string |
| ------------- | ---------------- |
| Ascii | +++++++++|||---- |
| ReducedAscii | ESEESEESEESE---- |
| ReducedModern | ESEESEESEESE──── |
| Markdown | EEE|||EEE|||ES-S |
| Modern | ┌┬┐├┼┤└┴┘│││──── |
| Fancy | ╭┬╮├┼┤╰┴╯│:│─−-⋅ |
| Blank | SSSSSSSSSSSSSSSS |
| Empty | EEEEEEEEEEEEEEEE |
For example, the string "ESEESEESEESE───"
is how the PreSet::ReducedModern
style is defined.
Defined in:
border.crConstant Summary
-
PREDEFINED_BORDERS =
{PreSet::Ascii => "+++++++++|||----", PreSet::ReducedAscii => "ESEESEESEESE----", PreSet::Modern => "┌┬┐├┼┤└┴┘│││────", PreSet::ReducedModern => "ESEESEESEESE────", PreSet::Markdown => "EEE|||EEE|||ES-S", PreSet::Fancy => "╭┬╮├┼┤╰┴╯│:│─−-⋅", PreSet::Blank => "SSSSSSSSSSSSSSSS", PreSet::Empty => "EEEEEEEEEEEEEEEE"}
-
PreSet
border definition hash constant
Constructors
-
.new(definition : String | PreSet = Config::Defaults.border_definition, styler : Styler = Config::Defaults.border_styler)
Creates a Border.
Constructor Detail
Creates a Border.
Optional (named) parameters, with default values:
-
definition: set of border connectors to use
Default value set byConfig::Defaults.border_definition
-
Styler: a user defined proc
Default value set byConfig::Defaults.border_styler
Examples :
border = Tablo::Border.new(Tablo::Border::PreSet::Fancy,
styler: ->(connectors : String) { connectors.colorize(:yellow).to_s })
or
border = Tablo::Border.new("┌┬┐├┼┤└┴┘│││────",
styler: ->(connectors : String) { connectors.colorize.fore(:blue).mode(:bold).to_s })
In general, a border is defined directly in table creation, as :
require "tablo"
table = Tablo::Table.new(["abc"],
border: Tablo::Border.new(:fancy)) do |t|
t.add_column("itself", &.itself)
end
puts table
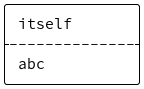