alias
Tablo::Cell::Data::Styler
Overview
The purpose of the styler is to apply stylistic effects to a previously formatted character string. For a terminal without graphic capabilities, these effects are limited to the use of color and/or character modes (bold, italic, etc.).
For cells of type Tablo::Cell::Data
(header and body), the styler Proc can take
5 different forms, as shown below by their commonly used parameter names and types:
- 1st form : (content :
String
) - 2nd form : (content :
String
, line_index :Int32
) - 3rd form : (value :
Tablo::CellType
, content :String
) - 4th form : (value :
Tablo::CellType
, coords :Tablo::Cell::Data::Coords
, content :String
) - 5th form : (value :
Tablo::CellType
, coords :Tablo::Cell::Data::Coords
, content :String
, line_index :Int32
)
The default styler vis defined byTablo::Config::Defaults.body_styler
(or
Tablo::Config::Defaults.header_styler
) and the return type is String for
all of them.
- content is the formatted value of the cell (after formatter
has been applied)
- line_index designates the line number in a (multi-line) cell (0..n).
These different forms can be used for conditional styling.
In a somewhat contrived example, we could write, using the 5th form:
require "tablo"
require "colorize"
table = Tablo::Table.new(["A", "B", "C"],
title: Tablo::Heading.new("My Title", framed: true),
body_styler: ->(_value : Tablo::CellType, coords : Tablo::Cell::Data::Coords, content : String, line_index : Int32) {
if line_index > 0
content.colorize(:magenta).mode(:bold).to_s
else
if coords.row_index % 2 == 0
coords.column_index == 0 ? content.colorize(:red).to_s : content.colorize(:green).to_s
else
content.colorize(:blue).to_s
end
end
}
) do |t|
t.add_column("itself", &.itself)
t.add_column("itself x 2", &.*(2))
t.add_column("itself x 3", &.*(3).chars.join("\n"))
end
puts table
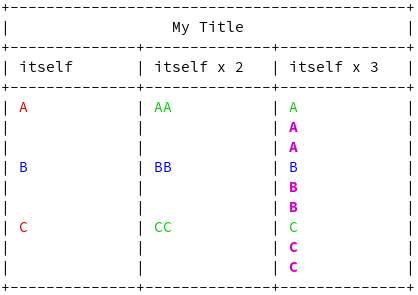
Or, more simply by using the 3rd form, to better differentiate between negative and positive values:
require "tablo"
require "colorize"
table = Tablo::Table.new([3.14, 2.78, 3.5],
title: Tablo::Heading.new("My Title", framed: true),
body_styler: ->(value : Tablo::CellType, content : String) {
if value.is_a?(Float64)
if value.as(Float64) < 0.0
content.colorize(:red).to_s
else
content.colorize(:green).to_s
end
else
content
end
}
) do |t|
t.add_column("itself", &.itself)
t.add_column("itself x 2", &.*(2).*(rand(10) < 5 ? -1 : 1))
t.add_column("itself x 3", &.*(3).*(rand(10) < 5 ? -1 : 1))
end
puts table
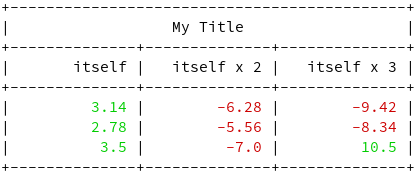
Alias Definition
String, Int32 -> String | String -> String | Tablo::CellType, String -> String | Tablo::CellType, Tablo::Cell::Data::Coords, String, Int32 -> String | Tablo::CellType, Tablo::Cell::Data::Coords, String -> String